티스토리 뷰
nestjs에서 api를 제대로 활용하기전에 데이터베이스를 연결해보자.
이번 프로젝트에서는 TypeORM을 활용하여 데이터베이스를 연결 및 생성한다.
TypeORM은 객체 모델과 관계형 모델간의 비효율적인 불일치를 해결하기위한 객체와 모델간의 매핑이 가능한 형태이다.
내용이 어렵다면 간단히, nestjs에서 테이블 생성 및 객체 연결까지 모두 진행한다고 이해하면 될 거 같다.
[준비 사항]
이번 프로젝트는 데이터베이스를 postgresql을 사용하였다, mysql과 90% 유사하지만 설치되는 라이브러리가 조금 다르다, mysql 도 함께 설명을 진행하겠다.
[설치]
-postgressql의 경우
$ npm install --save typeorm pg psql @nestjs/typeorm
-mysql의 경우
$ npm install --save typeorm mysql @nestjs/typeorm
-testModule 생성
$ nest g mo test
[엔티티 생성] photo.entity.ts
import { Entity, Column, PrimaryGeneratedColumn } from 'typeorm'; @Entity() export class Photo { @PrimaryGeneratedColumn() id: number; @Column({ length: 500 }) name: string; @Column() description: string; @Column() filename: string; @Column() views: number; @Column() isPublished: boolean; }
설명 - @Entity 어노테이션을 추가하여 Photo 엔티티를 생성
@Column 어노테이션을 추가하여 각각의 컬럼 생성
[AppModule.ts에 엔티티관련 내용 추가]
import { Module } from '@nestjs/common'; import { TypeOrmModule } from '@nestjs/typeorm'; import { TestModule } from './test/test.module'; import { Photo } from './test/entities/photo.entity'; @Module({ imports: [ TypeOrmModule.forRoot({ type: 'postgres', host: '127.0.0.1', port: 5432, username: 'postgres', password: '1', database: 'testApi', synchronize: true, logging: true, entities: [Photo], }), TestModule, ], }) export class AppModule {}
설명 - 127.0.0.1 서버의 id:postgres pw:1 db:testApi를 가진 환경의 데이터베이스 및 Photo 엔티티를 추가
[testModule에 엔티티관련된 내용 추가]
import { Module } from '@nestjs/common'; import { TypeOrmModule } from '@nestjs/typeorm'; import { Photo } from './entities/photo.entity'; import { TestController } from './test.controller'; import { TestService } from './test.service'; @Module({ imports: [TypeOrmModule.forFeature([Photo])], providers: [TestController, TestService], }) export class TestModule {}
[testService 엔티티관련 내용 추가]
import { Injectable } from '@nestjs/common'; import { InjectRepository } from '@nestjs/typeorm'; import { Repository } from 'typeorm'; import { Photo } from './entities/photo.entity'; @Injectable() export class TestService { constructor( @InjectRepository(Photo) private readonly repository: Repository<Photo>, ) {} getTest(): string { return 'test'; } }
[실행 확인] shell에서 테이블이 정상적으로 생성되어, 실제 데이터베이스에서 확인이 가능하다.
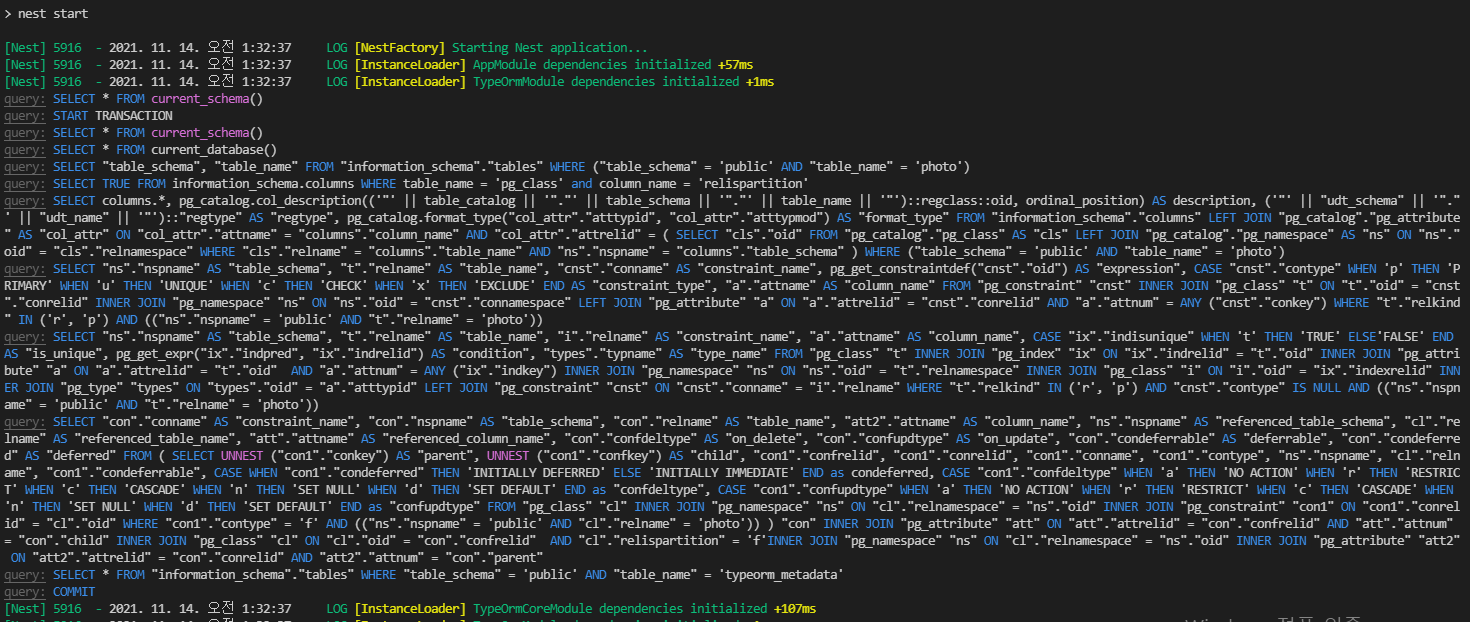
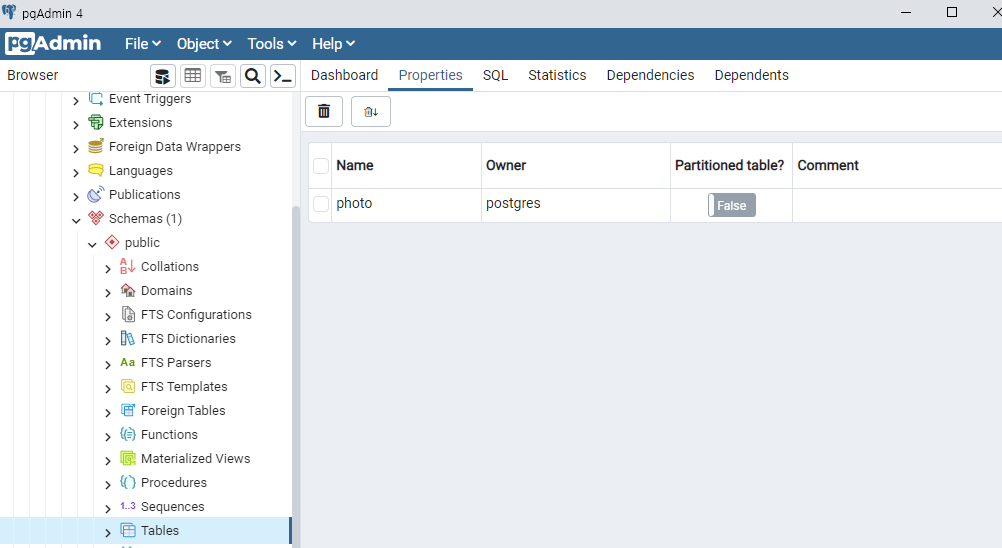
[commit 및 코드]
commit
https://github.com/gofogo2/nest-js-api/commit/9d15acdd1139c48a17dd20f5e96470973db5f97b
add database with typeorm · gofogo2/nest-js-api@9d15acd
Permalink This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository. Showing 8 changed files with 2,027 additions and 136 deletions. +1,965 −95 package-lock.json +5 −1 package.json +0 −21 src/app
github.com
code
https://github.com/gofogo2/nest-js-api.git
GitHub - gofogo2/nest-js-api
Contribute to gofogo2/nest-js-api development by creating an account on GitHub.
github.com
#nestjs #typescript #javascript #js #ts #api #controller #service #typeorm #database #mysql #postgres #sql #nestjs to db
'nest api 서버 개발 프로젝트' 카테고리의 다른 글
[nestjs] 4. nestjs환경에서 설정파일 config로 빼기(Joi, config, cross-env) (0) | 2021.11.15 |
---|---|
[nestjs] 3. nestjs 환경에서 기본적인 CRUD(Create Read Update Delete) 완성하기 (0) | 2021.11.14 |
[nestjs] 1. testController / testService 생성 및 helloworld (0) | 2021.11.14 |
[nestjs] 0. 프로젝트 생성 (0) | 2021.11.14 |
[typescript(타입스크립트)/nestjs]nestjs로 api 서버 1분만에 만들기 (0) | 2021.11.13 |